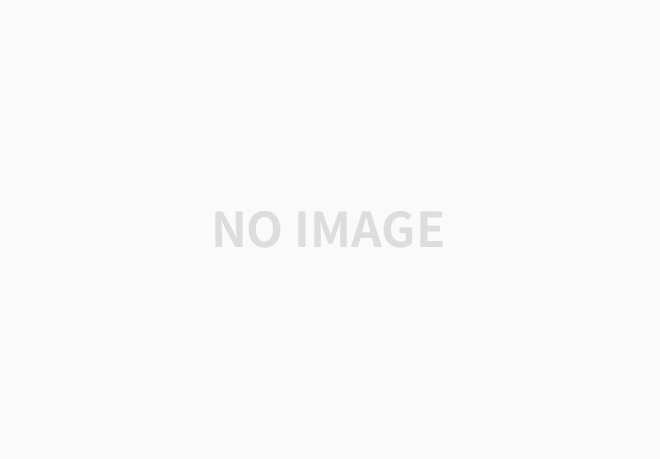
solution
1. bfs이용 (6방향 → 3차원 배열)
# 6593, 상범빌딩
import sys
from collections import deque
dx = [-1, 1, 0, 0, 0, 0]
dy = [0, 0, -1, 1, 0, 0]
dz = [0, 0, 0, 0, -1, 1]
def bfs(z, x, y):
visited[z][x][y] = 1
q = deque()
q.append((z, x, y))
while q:
c, a, b = q.popleft()
for i in range(6):
nz = c + dz[i]
nx = a + dx[i]
ny = b + dy[i]
if 0 <= nz < L and 0 <= nx < R and 0 <= ny < C:
if visited[nz][nx][ny] == 0 and sb[nz][nx][ny] != '#':
visited[nz][nx][ny] = visited[c][a][b] + 1
q.append((nz, nx, ny))
if sb[nz][nx][ny] == 'E':
return visited[c][a][b]
return -1
while True:
L, R, C = map(int, sys.stdin.readline().split())
if L == 0 and R == 0 and C == 0:
sys.exit()
sb = []
visited = [[[0] * C for _ in range(R)] for __ in range(L)]
for z in range(L):
b = []
for x in range(R):
b.append(list(sys.stdin.readline().rstrip()))
sb.append(b)
input()
for z in range(L):
for x in range(R):
for y in range(C):
if sb[z][x][y] == 'S':
res = bfs(z, x, y)
if res == -1:
print('Trapped!')
else:
print('Escaped in', res, 'minute(s).')
'알고리즘 > DFS | BFS' 카테고리의 다른 글
파이썬 | 백준 | 1743 | 음식물 피하기 (0) | 2020.10.04 |
---|---|
파이썬 | 백준 | 2458 | 키 순서 (0) | 2020.09.29 |
파이썬 | 백준 | 2606 | 바이러스 (0) | 2020.09.02 |
파이썬 | 백준 | 2644 | 촌수계산 (0) | 2020.08.31 |
파이썬 | 백준 | 5567 | 결혼식 (0) | 2020.08.28 |